What is an abstract class and how to implement an abstract class in Java?
Abstract class in Java! Being a developer/Automation Engineer you must come across the word Abstract class or abstraction. Even it’s one of the favorite topics for interviewers and they try to check your knowledge on abstraction. So this tutorial will help you to understand what is abstract? when to use abstraction or abstract class? and step-by-step implementation of abstraction in Java.
What is Abstract Class?
An abstract class is a class that needs the keyword “abstract” for declaration. It may or may not be abstract methods. We can create subclasses from an abstract class.
Sample abstract Class declaration
package abstractDemo; public abstract class Base { }
What is Abstract Method?
In abstract class we declare a method with the abstract keyword (without braces and followed by a semicolon);
package abstractDemo; public abstract class Base { abstract void sum(int a, int b); abstract void subtract(int a, int b); }
The subclass implements these methods.
Note:
Abstract Class can have a mixture of abstract methods and non-abstract methods.
package abstractDemo; public abstract class Base { abstract void sum(int a, int b); abstract void subtract(int a, int b); public void displayMessage() { System.out.println("This is non abstract method"); } }
When to use Abstract Class?
Generally, an abstract class is to create a base class that can extend by a subclass to create a full implementation. We use abstract Class basically for:
- Sharing Code among several closely relate classes.
- Declaring common methods and implementing them in abstract classes. We use these methods in the subclass.
- Abstract methods and fields can be static and non-static fields. This enables you to define methods that can access and then modify the state of the object in which they belong.
Example:
Let’s assume you have to develop a calculator and calculator functionalities (methods) are taken into use by different vendors with little modification. So it will be a great idea to create an abstract class and declare all the methods in the abstract class. And during each vendor class calculator methods can be implemented as per their need. This is one of the best use cases to understand the abstract class.

Step by Step Implementation of Abstract Class:
To explain abstract class, here is the step-by-step implementation of abstract class and their subclass. It will give a complete understanding. Refer below for step by step implementation and code explanation:
Step 1:
Open Java Project in Eclipse and create an abstract class:
- Java should be installed and Eclipse or IntelliJ Idea should be set up. (If Java is not installed then refer to our tutorial https://thoughtcoders.com/blog/install-java-and-setup-environment-variable/
- Java Project should be created
- Step 1: Open Java Project in Eclipse and create an abstract class
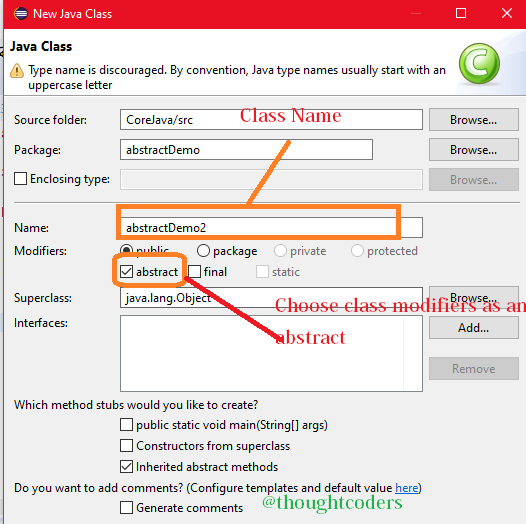
package abstractDemo; public abstract class Base { }
Step2:
Create Abstract Methods: Now you can declare abstract methods within the above abstract class. Abstract methods are a method without implementation.
package abstractDemo; public abstract class Base { abstract void sum(int a, int b); abstract void subtract(int a, int b); public void displayMessage() { System.out.println("This is non abstract method"); } }
Step 3:
Write Subclass (Child Class) and extends abstract Class: To use the parent class method we need to inherit parent class by keywords “extends”. Refer below
package abstractDemo; public class Childclass extends Base { public static void main(String[] args) { } }
Step 4:
Implement abstract class methods:

Step 5:
Override annotation used at the time of definition:
public class Childclass extends Base {
package abstractDemo; public class Childclass extends Base { public static void main(String[] args) { } @Override void sum(int a, int b) { } @Override void subtract(int a, int b) { } }
Step 6:
package abstractDemo; public class Childclass extends Base { public static void main(String[] args) { // Make object of Child Class to use implemented abstract class Childclass child = new Childclass(); child.sum(3,4); child.subtract(5,1); // method which implemented in Base class child.displayMessage(); } @Override void sum(int a, int b) { int c = a+ b; System.out.println("Sum method implmented here: "+c); } @Override void subtract(int a, int b) { int c = a- b; System.out.println("subtract method implmented here: "+c); } }
Console Output:

Brief Summary:
- We declare an abstract class with the keyword “abstract”.
- Java can’t instantiate an abstract class.
- An abstract class with the private and final method is not possible in Java.
- An abstract class with zero abstract methods is possible.
- The static method should have a compulsory implementation available while abstract methods should not have the definition. So the static method is not possible in the abstract class.
- If all methods of an abstract class are abstract then better to make a class Interface.
- The subclass is responsible for the implementation of abstract methods.
- Abstract Classes should not be static, final, define public, protected, and private concrete methods.
With the result that now you know how to use abstract classes. Hope you enjoyed this article and understand the abstract and are able to implement it in your framework. To sum up, to learn more about other topics refer to our next blog post. If you have any doubt then feels free to contact us at query@thoughtcoders.com
To that end, for more information on this, join us on Facebook and LinkedIn!
[mailpoet_form id=”2″]