What is Scenario Outline and Example in Cucumber BDD Frameworks
Data-Driven testing is a very genuine expectation from any automation framework. With this purpose in mind, to perform data-driven testing in the BDD framework we need to use scenario outline and examples. With attention to the automation community, we created this tutorial.
In this tutorial, we will learn about Scenario Outline and Examples. It gives us the flexibility to perform data-driven testing in BDD Framework. In simpler words it allows us to perform the same test over multiple data.
This tutorial explains what is Scenario Outline an example, why we use and how to write code. Also, each step is explained in detail by the snapshot.
Scenario Outline
It is Gherkin Keyword that is used in feature file writing, used to perform the same scenario testing on the different data sets, it must have the “Examples” keyword and in the example, we pass test data.
Sample feature File:
Feature: Test Login feature with multiple credentials @AB1 Scenario Outline:TestCase:"<TCID>"Test Newtour website with Multiple credentials Given Open the browser and open application When user enter "<username>" and "<password>" Then user should be successfully login into Application Examples: | TCID | username | password | | TC1 | mercury | mercury | | TC2 | mercury | mercury | | TC3 | mercury | mercury | | TC4 | mercury | mercury |
In the above feature file, perform a login scenario for four users (mercury, mercury1, mercury2, and mercury3). For example, we passed user details ie username, and password.
Prerequisite: For this tutorial, you have to do the following setup
- Java and IDE should be set up on the machine (If Java is not installed then click on this link)
- Set up cucumber BDD Project. ( To create the first Cucumber project click on this link)
Go to feature folder, create new feature file and write scenario with scenario outline.
Feature: Test Login feature with multiple credentials @AB1 Scenario Outline:TestCase:"<TCID>"Test Newtour website with Multiple credentials Given Open the browser and open application When user enter "<username>" and "<password>" Then user should be successfully login into Application Examples: | TCID | username | password | | TC1 | mercury | mercury | | TC2 | mercury | mercury | | TC3 | mercury | mercury | | TC4 | mercury | mercury |
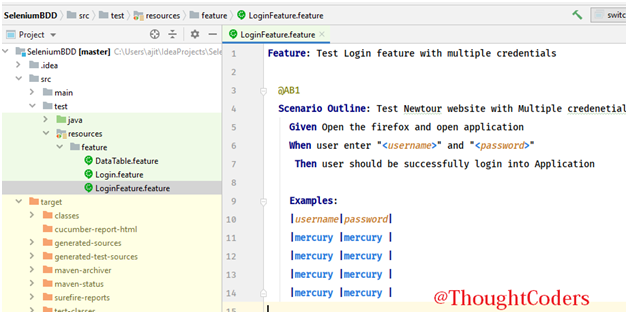
Write test data and Examples and map steps variable using “<username>” and “<password>”.
Implement scenario steps in the step definition file
package stepDef; import cucumber.api.PendingException; import cucumber.api.java.en.Given; import cucumber.api.java.en.Then; import cucumber.api.java.en.When; import io.github.bonigarcia.wdm.WebDriverManager; import org.junit.Assert; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class stepDefs { public WebDriver driver=null; @Given("^Open the browser and open application$") public void OpenBrowser(){ WebDriverManager.chromedriver().version("83.0.4103.61").setup(); driver = new ChromeDriver(); driver.get("http://demo.guru99.com/test/newtours/index.php"); driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); } @When("^enter the Username and Password$") public void enter_the_Username_and_Password() throws Throwable { driver.findElement(By.xpath("//input[@name='userName']")).sendKeys("mercury"); driver.findElement(By.xpath("//input[@name='password']")).sendKeys("mercury"); driver.findElement(By.xpath("//input[@name='login']")).click(); } @When("^user enter \"(.*)\" and \"(.*)\"$") public void userEnterUsernameAndPassword(String username, String password) throws Throwable { driver.findElement(By.xpath("//input[@name='userName']")).sendKeys(username); driver.findElement(By.xpath("//input[@name='password']")).sendKeys(password); driver.findElement(By.xpath("//input[@name='login']")).click(); } @Then("^user should be successfully login into Application$") public void user_should_be_successfully_login_into_Application() throws Throwable { Assert.assertEquals("Login: Mercury Tours",driver.getTitle()); driver.close(); } }
Open TestRunner Class and pass scenario tag and run.
tags={"@AB1"},
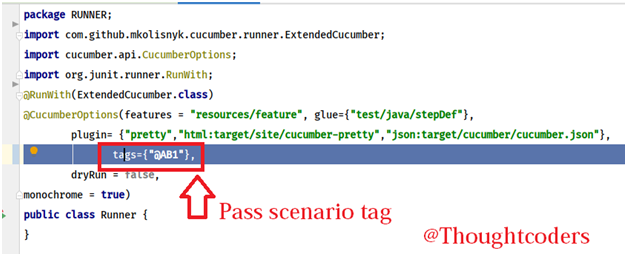
Complete Runner Class Code:
import cucumber.api.CucumberOptions; import cucumber.api.junit.Cucumber; import org.junit.runner.RunWith; @RunWith(Cucumber.class) @CucumberOptions( features = {"src/test/resources/features"}, glue = {"stepDef"}, tags={"@AB1"}, plugin = {"json:target/cucumber.json"}) public class TestRunner { }
Run runner class and verify the result.

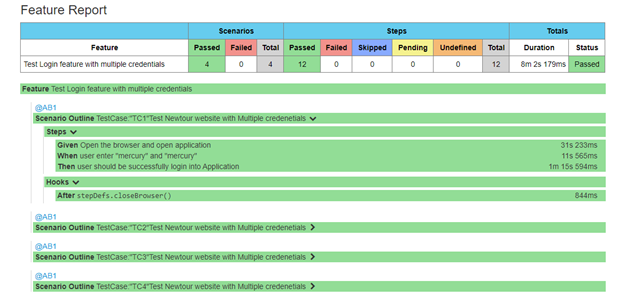
Hope you have followed the above steps and successfully implemented examples in your BDD framework. If you face any issue then feel free to write us query@thoughtcoders.com.