As the world is evolving at a rapid pace and especially in the field of software development, testing plays a critical role in making the process defect or bug-free. First thing to remember, Selenium is one such tool that lets us automate the whole process of manual testing & that we term as Automation Testing. And, this article is on How to handle multiple windows using selenium.
What is Selenium WebDriver?
Selenium is a web automation tool which provides testing framework to automate the testing process of web applications. One can write test script in selenium in many programming languages like- Java Python, C# etc.
Handling Multiple Windows In Selenium
During testing, we find many scenarios where multiple windows are opened due to some events like click, hover, refresh, or some other business logic. So, we need to manage and handle multiple windows in automation. In other words, fortunately, Selenium provides some very robust methods like- switchToWindow, getWindowHandle, and getWindowHandles which helps us to handle multiple windows.
So, now move on to some illustration part where we will learn how we can write automation script for handling multiple windows using selenium
Example:
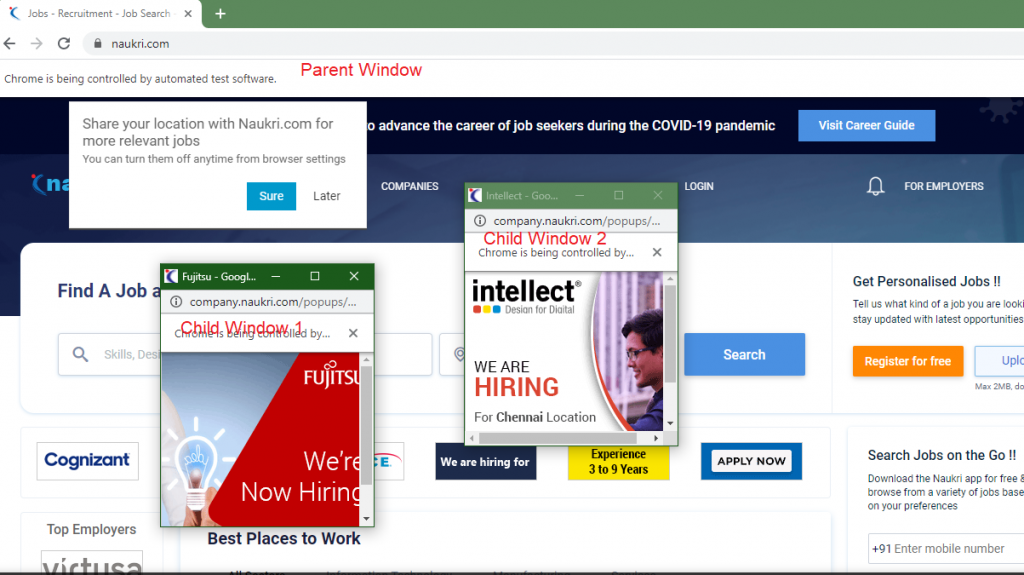
The above Image is of Naukri.com with multiple windows opened. Now, Refer below-mentioned steps to write a script for multiple windows handling:
Pre-requisite:
- Selenium Project should be created. (If selenium is not set up on your system then, read out this tutorial– Selenium Installation Tutorial With Example)
Steps to Follow:
- Step 1: Open Selenium Project and create one java class to write code/script
- Step 2: Create one main method and write code to open browser and navigate to URL
Code for Step 1 & Step 2:
public static void main(String[] args) throws InterruptedException {
// Set true to turn off ChromeDriver log on Console
System.setProperty("webdriver.chrome.silentOutput", "true");
// Set ChromeDriver Path
System.setProperty("webdriver.chrome.driver", "C:\Users\user\Documents\SeleniumDrivers\chromedriver_win32\chromedriver.exe");
// Make instance of ChromeDriver
WebDriver driver = new ChromeDriver();
// Maximize Browser
driver.manage().window().maximize();
// Set implicit wait 30 seconds to wait for WebElement
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
// Navigate to URL a
driver.get("https://www.naukri.com");
// Wait 8 seconds so that all backend Java script executed
Thread.sleep(8000);
}
- Step 3: Add Code to get parent window handle and print current widow title
Code for Step 3:
//Code to get parent Widow Handle
String parentWindow = driver.getWindowHandle();
// Print Current Window Title
System.out.println("Parent Window Name: "+driver.getTitle());
- Step 4: Use Methods getWindowHandles() to get all the windows in Set Collections. WindowHandle is unique identifier which remains constant through session.
Code for Step 4:
Set openWindows = driver.getWindowHandles();

Above Image description: Itellij IDE– Debugger Mode showing windows ID.
Selenium methods for Handling Multiple Windows:
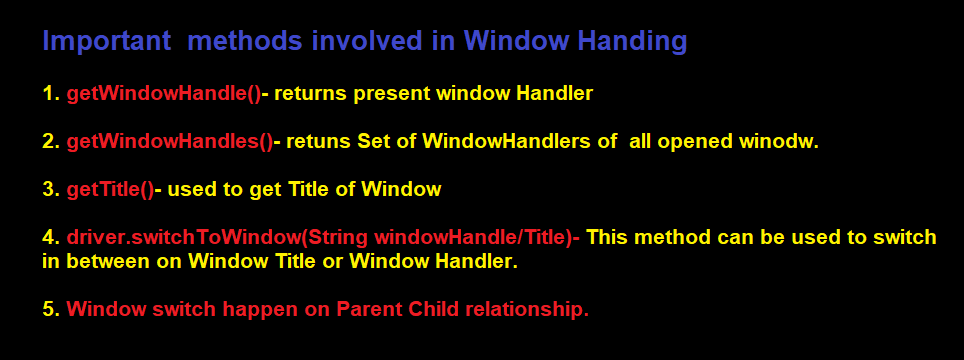
- Step 5: Iterate Set and use method switchToWindow(String handler) to switch to a specific window. In the below code driver switches among all the open windows and print titles.
Code for Step 5:
for (String winTitle : openWindows) {
System.out.println("Win Title: " + driver.switchTo().window(winTitle).getTitle());
}
// Again switch to parent window and print title
driver.switchTo().window(parentWindow);
System.out.println("Parent Window: " + driver.getTitle());
driver.quit();
- Step 6: Set can be iterated using Iterator. Refer below code:
Code for Step 6:
Iterator win = openWindows.iterator();
while (win.hasNext()) {
String tempWin = win.next();
if (!tempWin.contentEquals(parentWindow)) {
System.out.println("\nChild Window: " + driver.switchTo().window(tempWin).getTitle());
}
}
driver.quit();
Automation Script to handle multiple windows using selenium
Complete Code: (Automation Script to handle multiple windows using selenium)
package stepDef;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
import java.util.Iterator;
import java.util.Set;
import java.util.concurrent.TimeUnit;
public class switchToWindow {public static void main(String[] args) throws InterruptedException {
System.setProperty("webdriver.chrome.silentOutput", "true"); System.setProperty("webdriver.chrome.driver", "C:\\Users\\user\\Documents\\SeleniumDrivers\\chromedriver_win32\\chromedriver.exe");
// WebDriverManager.chromedriver().version("80.0").setup();
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); driver.get("https://www.naukri.com"); Thread.sleep(7500);
String parentWindow = driver.getWindowHandle();
System.out.println("Parent Window Name: " + driver.getTitle()); //getWindowHandles() method returns all the open windows.
Set<String> openWindows = driver.getWindowHandles();
System.out.print("All the open windows handles: " + openWindows);
for (String winTitle : openWindows) {
System.out.println("Win Title: " + driver.switchTo().window(winTitle).getTitle());
}
driver.switchTo().window(parentWindow);
System.out.println("Parent Window: " + driver.getTitle());
System.out.println("******************use iterator to iterate over window***************");
Iterator<String> win = openWindows.iterator();
while (win.hasNext()) {
String tempWin = win.next();
if (!tempWin.contentEquals(parentWindow)) {
System.out.println("\nChild Window: " + driver.switchTo().window(tempWin).getTitle());
}
}
driver.quit();
}
}
Steps Summary:
Summing up
1. Open Browser and Navigate to URL
2. Click on elements (like- button, hyperlink, etc.) to open Multiple windows open.
3. Get Parent Window Handler and store in a variable which we will use in further/
4. Get all the Window Handlers and store them into a set.
5. Lastly, Iterate over all window Handlers and switch to the desired window as per your test case.
Congratulations! You have completed your automation script for window switch and using above concept you can handle multiple windows at a time and switch in desired window. Hope this article helps you.
To this end, Feel free to write your feedback/challenges on query@thoughtcoders.com
[mailpoet_form id=”2″]